Would you like to make this site your homepage? It's fast and easy...
Yes, Please make this my home page!
Concurrent Programming with Java
Lab Manual, Version 1.0, F. Astha Ekadiyanto,
2002.
[Contents] [Next] [Previous]
Lab 1: Introduction to Lab. Environment and Object Orientation
Msc Class
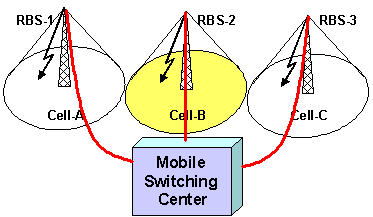
Mobile Switching Centre is the central of the mobile network. It registers
all the RBS and their corresponding coverage. All management issues in the mobile
network are served by MSC. This means MSC responsible for all services to mobile
stations and allocate the best Rbs to serve a certain location. The Msc should
be able to access any Rbs objects in its record and also the Cell objects in
the corresponding Rbs. This communication will ensure the capability of an Msc
to provide services.
In this Lab, a simplified Msc is defined as follows:
What an MSC should have?
- Of course an array of RBS objects to store all the registered Rbs references.
- Capacity of MSC in terms of number of RBS objects
What an MSC should behave?
- Register all the RBSs into the array.
- Finding RBS candidates to serve a certain point of access given by x,y coordinate.
- Find RBS with a certain ID for administrative purposes since an Rbs considered
to have a unique ID.
- Show RBS information and its neighbors (to provide simple debugging on how
the service provided. The output will be displayed in console).
These definitions can be implemented in Java Language as follows :
File name: Msc.java
Class Fields :
- MAXRBS : a constant used to limit the Msc record capacity
to a maximum number of Rbs.
- numOfRbs : indicates the total number of Rbs registered
in the Msc.
- rbss : array of Rbs that stores all the Rbs records (references).
Class Constructors: There are no class constructor defined (but a primitive
constructor will be implemented implicitly)
Class Methods:
- addRbs(Rbs ) : registers the Rbs argument reference into
the rbss array.
- isRegistered(Rbs) : check whether an Rbs exists in the
rbss array to prevent duplicates.
- setNeighboring(Rbs,Rbs) : set two Rbs as neighbors (inform
both Rbs that each other is neighboring)
- findRbs(x,y) : Find an Rbs that can serve a certain point
(x, y coordinate)
- findRbs(String) : A polymorph of findRbs but with different
function that is to find an Rbs in the rbss array that has the same ID as
the String argument.
- showNeighbors(Rbs) : Prints out all the Rbs' neighbors
(access the Rbs' Enumeration list).
import java.util.Enumeration; //Required because Enumeration Class is used in the class /** * Mobile Switching Center * @author CPwJ * @version 1.0 (2002) */ public class Msc { private static final int MAXRBS=100; //Maximum RBS to be registered private int numOfRbs=0; //Number of RBS currently registered private Rbs[] rbss=new Rbs[MAXRBS]; //For storing RBS reference /** * Register an Rbs into Msc * @param Rbs the Radio Base Station to be registered */ public void addRbs(Rbs station) { if ( (numOfRbs < MAXRBS) && !(isRegistered(station)) ) { rbss[numOfRbs++] = station; } } private boolean isRegistered(Rbs station) { for (int x=0; x<numOfRbs; x++) { if ( rbss[x]==station) return true; } return false; } /** * Set two Rbs as neighbors * @param Rbs1 First neighboring Radio Base Station * @param Rbs2 Second neighboring Radio Base Station */ public static void setNeighboring(Rbs station1, Rbs station2) { station1.addNeighbor(station2); station2.addNeighbor(station1); } /** * Find an Rbs that covers a point of access * @param x the point of access coordinate * @param y the point of access coordinate */ public Rbs findRbs(double x, double y)
{ for (int i=0; i<numOfRbs; i++) { if ( rbss[i].getCell().coverage(x,y)) return rbss[i]; } return null; } /** * Find an Rbs with an Identification string * @param id the Identification String */ public Rbs findRbs(String id)
{ for (int i=0; i < numOfRbs; i++) { if ( id.equals(rbss[i].getID()) ) return rbss[i]; } return null; } /** * List all the neighbors of an Rbs * @param Rbs the Rbs in the center */ public static void showNeighbors(Rbs station) { System.out.println("Neighboring RBS are:"); station.resetElements(); for ( Enumeration e = station; e.hasMoreElements();) System.out.println(e.nextElement()); } }
|
[Contents] [Next] [Previous]