Would you like to make this site your homepage? It's fast and easy...
Yes, Please make this my home page!
Concurrent Programming with Java
Lab Manual, Version 1.0, F. Astha Ekadiyanto,
2002.
[Contents] [Next] [Previous]
Lab 1: Introduction to Lab. Environment and Object Orientation
Rbs Class
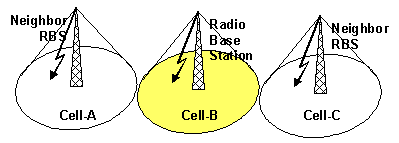
The Rbs Class is an individual Class (only extends Object Class) to
represent the Radio Base Station entity in the Mobile Network. An RBS is a gateway
for Mobile Stations (or Mobile Users) to connect to the Mobile Network.
An RBS should only serve one cell (thus RBS should have a Cell object).
In order to use an RBS, one should register them in their MSC. This means
that RBS should serve all the inquiries demanded by MSC.
To help defining RBS classification, a simple demonstration of properties and
behaviour is performed here.
What an RBS should have?
- A Cell being served
- RBS ID
- Records of Neighbor RBSs (stored in an array or Rbs).
What an RBS should behave?
- Provide information about the Cell being served
This behaviour is simply returns the reference to the serviced Cell so that
any objects can directly communicate to the Cell, but not changes the Cell's
reference stored in the Rbs object (Think as if it is a read only behaviour)
- Register neighboring RBSs
This will enables other objects to communicate with the Rbs to ask it to register
its neighboring Rbs. This behaviour is necessary by the Msc to be able
to setup a Mobile Network topology.
- Enable other objects to query all the registered neighboring RBSs
In some cases, an Msc needs to query all the registered neighbors of
an Rbs to find better service quality for a Mobile Station. Java API suggests
an interface called Enumeration to classify enumerated behaviour of
an object. Thus, any classes that implements Enumeration should implement
the two methods so called hasMoreElements() and nextElement().
These definitions can be implemented in Java Language as follows :
File name: Rbs.java
Class Fields :
- MAXNEIGHBOR : A constant represents the maximum number
of neighbors that an Rbs can register.
- numOfNeighbor : A field that indicates the number of neighbor
currently registered
- cellAssigned: A field to refer to the cell assigned to
be served
- id: A String field that represents the Rbs Identification
String
- neighborRbs: An array of Rbs that stores all the references
of registered neighboring Rbs.
- current: This field is a pointer used for enumeration when
other objects queries the registered Rbs.
Class Constructors:
- Rbs() : Initializes all Class Fields without arguments
- Rbs(String id) : Initializes all Class Fields and set Rbs
Identification String.
- Rbs(String id, Cell) : Initializes all Class Fields and
set Rbs ID and registers serviced cell.
Class Methods:
- setID(String) : set the Rbs ID.
- setCell(Cell) : set the reference of Cell serviced by
the Rbs.
- getID() : get the Rbs ID.
- getCell() : get the reference to the Cell serviced by
the Rbs.
- toString() : returns the String representation of this
Rbs (used in direct String operation).
- addNeighbor(Rbs) : registers the Rbs argument as one of
the Rbs neighbors.
- isNeighbor(Rbs) : check whether the Rbs argument is registered
as neighbor.
- resetElements() : reset the current pointer for Enumeration
of the neighboring Rbs.
- hasMoreElements() : implements the Enumeration method
to check is there are more elements.
- nextElement() : implements the Enumeration method to return
the next element.
import java.util.*; /** * Radio Base Station * * @author CPwJ * @version 1.0 (2002) */ public class Rbs implements Enumeration //Enumeration is an interface defined in Java API { // instance variables private static final int MAXNEIGHBOR=6; //Maximum number of neighbors private int numOfNeighbor=0; //Number of neighbor currently registered private Cell cellAssigned; //The cell assigned to be served private String id; //The RBS identification String private Rbs[] neighborRbs=new Rbs[MAXNEIGHBOR]; //The array of neighbors private int current = 0; //The pointer to neighbor index (Enumeration interface)
/**
* Rbs Constructor
*/
public Rbs()
{
setID("Unidentified");
setCell(null);
}
public Rbs(String id)
{
setID(id);
setCell(null);
}
public Rbs(String id, Cell servicecell)
{
setID(id);
setCell(servicecell);
}
/**
* Change the Rbs Identification string
* @param ID Identification String
*/
public void setID(String id)
{
this.id = id;
}
/**
* Change the Rbs Cell assignment
* @param servicecell The Cell assigned to the Rbs
*/
public void setCell(Cell servicecell)
{
cellAssigned = servicecell;
}
/**
* Read the Rbs Identification String
* @return String ID, Rbs Identification
*/
public String getID()
{
return id;
}
/**
* Get the Cell assigned to this Rbs
* @return The Cell served by this Rbs
*/
public Cell getCell()
{
return cellAssigned;
}
/**
* Regiters all neighboring Rbs
* @param Rbs The neighboring RBS
*/
public void addNeighbor(Rbs neighbor)
{
if ((neighbor!=this) && (numOfNeighbor<MAXNEIGHBOR) && !(isNeighbor(neighbor)) )
{
neighborRbs[numOfNeighbor++] = neighbor;
}
}
private boolean isNeighbor(Rbs neighbor)
{
for (int x=0; x<numOfNeighbor; x++)
{
if ( neighborRbs[x]==neighbor ) return true;
}
return false;
}
/**
* Represent the Rbs content information
* @return String RBS Information
*/
public String toString()
{
return "RBS ID:"+ getID() + ", " + getCell();
}
/**
* Reset the pointer of neighbor Rbs element enumeration
*/
public void resetElements()
{
current=0;
}
/**
* Check if there are more neighbor Rbs element in the enumerated list
* @return True if the RBS has been registered as neighbor
* @return False if the RBS has been registered as neighbor
*/
public boolean hasMoreElements()
{
if (current < numOfNeighbor)
return true;
else
return false;
}
/**
* Get the next neighbor Rbs element in the enumerated list
* @return Rbs reference of the next registered neighbor
*/
public Object nextElement()
{
if (hasMoreElements())
return neighborRbs[current++];
return null;
}
}
|
[Contents] [Next] [Previous]