Would you like to make this site your homepage? It's fast and easy...
Yes, Please make this my home page!
Concurrent Programming with Java
Lab Manual, Version 1.0, F. Astha Ekadiyanto,
2002.
[Contents] [Next] [Previous]
Lab 1: Introduction to Lab. Environment and Object Orientation
HexCell Class
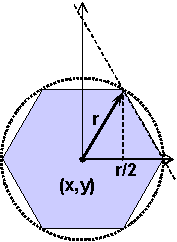
The Hex Cell Class is a subclass of Cell Class.
It is a specialized cell which only handles hexagonal shape cells.
Thus, there should be some overrides in the class definition to make
it more specialized.
The special classification of a Hex Cell Class is as follows:
- Calculate its area using Hexagonal equation which is
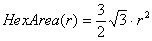
- Override the coverage behavior since the super class definition is not valid
for hex cells.
The overide can be performed by transforming the relative location of x,y
point into the first quardrat of the Hexagonal area and then check whether
it exceeds the boundaries.
These definitions can be implemented in Java Language as follows :
File name: HexCell.java
Class Fields :
- CELLTYPE : Indicates the type of shape it handles.
- RATIO : A constant used in area calculation.
Class Constructors:
Class Methods:
- area( ) : returns the area of the Cell (implements which
was once abstract in superclass).
- coverage(x,y) : returns true when x,y is covered in the
Cell (overrides superclass behavior)
- yLimit(x) : returns the first quardrant of y-boundary
by given relative x (only used in coverage(x,y) method - it
is private).
import java.lang.Math;
// Required by Math.sqrt() static method call in the class definition
/**
* A Circle Cell
*
* @author: CPwJ
* @version: 1.0 (2002)
*/
public abstract class HexCell extends Cell
{
// instance variables
//Information about a cell (x,y coordinate and r cell radius)
private static final double RATIO = 2.5980762; //ratio used in area calculation private static final String CELLTYPE = "Hexagonal"; //type of cell shape /** * CircleCell constructor * @param x Cell X coordinate * @param y Cell Y coordinate * @param r Cell Radius */ public HexCell(double x, double y, double r) { super(x,y,r); } /** * Calculate the area of a Circle shape Cell * @return the area of the Circle Cell */ public double area() { return RATIO*getR()*getR(); }
/** * Check whether a point is covered by the Hex cell * @param x The x point of access coordinate * @param y The y point of access coordinate * @return True/False */ public boolean coverage(double x, double y) { double relativeX = Math.abs(x-getX()); double relativeY = Math.abs(y-getY()); if ( relativeY > yLimit(relativeX) ) return false; return true; } /** * Calculate the first quardrant of Hex boundary * @param x The x point of access coordinate * @return The Y boundary */ private double yLimit(double x) { double r = getR(); double maxY = r * Math.sin(Math.PI / 3); double minY = 0.0; if ( x < r/2 ) return maxY; if ( x > r ) return minY; return (2 * maxY * (1 - x / r) ); } }
|
[Contents] [Next] [Previous]