Would you like to make this site your homepage? It's fast and easy...
Yes, Please make this my home page!
Concurrent Programming with Java
Lab Manual, Version 1.0, F. Astha Ekadiyanto,
2002.
[Contents] [Next] [Previous]
Lab 1: Introduction to Lab. Environment and Object Orientation
SquareCell Class
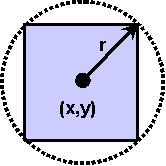
The SquareCell Class is a subclass of Cell Class.
It is a specialized cell which only handles square shape cells.
Thus, there should be some overrides in the class definition to make
it more specialized.
The special classification of a SquareCell Class is as follows:
- Calculate its area using Square equation which is simply:
- Override the coverage behavior since the super class definition is not valid
for square cells.
These definitions can be implemented in Java Language as follows :
File name: SquareCell.java
Class Fields :
- CELLTYPE : Indicates the type of shape it handles.
- RATIO : A constant used in area calculation.
Class Constructors:
Class Methods:
- area( ) : returns the area of the Cell (implements which
was once abstract in superclass).
- coverage(x,y) : returns true when x,y is covered in the
Cell (overrides superclass behavior).
import java.lang.Math; /** * A SquareCell * * @author CPwJ * @version 1.0 (2002) */ public class SquareCell extends Cell { private static final double RATIO = 2; //ratio used in area calculation private static final String CELLTYPE = "Square"; //type of cell shape /** * SquareCell constructor * @param x Cell X coordinate * @param y Cell Y coordinate * @param r Cell Radius */ public SquareCell(double x, double y, double r) { super(x,y,r); } /** * Calculate the area of a Square shape Cell * @return the area of the Square Cell */ public double area() { return RATIO*getR()*getR(); } /** * Check whether a point is covered by the Square cell * @param x The x point of access coordinate * @param y The y point of access coordinate * @return True/False */ public boolean coverage(double x, double y) { double xyLimit = getR()/Math.sqrt(2.0); if ((Math.abs(x-getX()) > xyLimit) || (Math.abs(y-getY()) > xyLimit)) return false; return true; } }
|
[Contents] [Next] [Previous]